tl;dr
- Trying to learn more
- Ran across a JavaScript feature proposal for Array.prototype.group()
- Peaked behind the curtain of the future of JavaScript by way of TC39
- Learned of core-js (‘npm install core-js’)
- Brought Array.prototype.group into my Nodejs module project via core-js (“import group from ‘core-js’;”)
- Played with Tomorrow’s JavaScript today!
Still want to read more? Great!
I’ve been a JavaScript developer…well…for about as long as I’ve been a developer at all. That said, and admittedly a little embarrassingly, I’m far from an expert on the language – but I’m learning more everyday, including more about how little I know.
As if often the case with developers, you complete school and you quickly realize that you need a job. Armed with a bunch of CS theory, and what’s basically a beginners level of experience, you pick up new skills and tricks as you need them along the way – but rarely is there sufficient time to learn much else outside of this while maintaining balance in other parts of your life…
This is the process for years for many/most developers – you show up for work, you’re given a feature to implement, you encounter something you don’t know how to do, you do a little research, you try a few things until you find something that works, and then you move on – rarely thinking about it again. As the years go on, you keep doing that thing that you found that worked – but rarely do most of us dig in deeper to understand more or to find alternatives. Again, there’s unfortunately only so many hours in a day, week, month, year, and lifetime – and there’s a lot to living outside of punching a keyboard.
But I digress… I’m trying to be better – I’m trying to be proactive… I’m trying to dig deeper, to gain a greater understanding and to perhaps learn some alternative approaches to old problems along the way.
In my recent adventures of funemployment, I decided to play around more free-form in JavaScript… I find the MDN Web docs to be an excellent resource for JavaScript, which is where I began my journey of playing with experimental JavaScript features/proposals today. I figured “let’s get back to the basics” and take a look at the standard built-in objects, which is when I ran across the experimental proposal Array.prototype.group() – that cute little experimental beaker icon just screamed out to me.
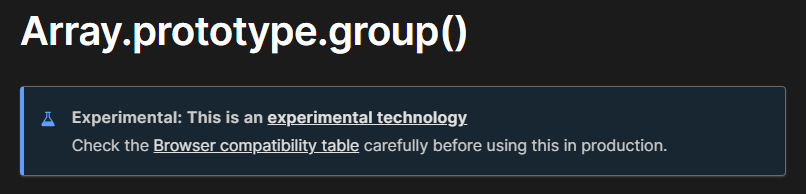
Consider the following…
const inventory = [
{ name: "asparagus", type: "vegetables", quantity: 5 },
{ name: "bananas", type: "fruit", quantity: 0 },
{ name: "goat", type: "meat", quantity: 23 },
{ name: "cherries", type: "fruit", quantity: 5 },
{ name: "fish", type: "meat", quantity: 22 },
];
const result = inventory.group(({ type }) => type);
/* Result is:
{
vegetables: [
{ name: 'asparagus', type: 'vegetables', quantity: 5 },
],
fruit: [
{ name: "bananas", type: "fruit", quantity: 0 },
{ name: "cherries", type: "fruit", quantity: 5 }
],
meat: [
{ name: "goat", type: "meat", quantity: 23 },
{ name: "fish", type: "meat", quantity: 22 }
]
}
*/
We have an array of JSON objects named ‘inventory’ – with standardized properties associated with each contained object. As we can see, calling array.group() passing in ‘type’ as our associative element – conceptually, we can think of this as a category. As such, we can see the resulting output of our group() call is an object comprised of our ‘type categories’, which are arrays containing the matching type objects.
I thought this was pretty cool and useful, so I wanted to try it out… Naively, I thought “Nodejs always has bleeding edge stuff – so let’s just plop in our playground code…”.
D:\w\nodejs\csv.js:45
jsonArr.group((country => country));
^
TypeError: jsonArr.group is not a function
at Object.<anonymous> (D:\w\nodejs\csv.js:45:9)
at Module._compile (node:internal/modules/cjs/loader:1159:14)
at Module._extensions..js (node:internal/modules/cjs/loader:1213:10)
at Module.load (node:internal/modules/cjs/loader:1037:32)
at Module._load (node:internal/modules/cjs/loader:878:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12)
at node:internal/main/run_main_module:23:47
Node.js v18.12.1
Whelp… That’s not going to get it – Array.prototype.group() doesn’t exist… Why? Because it’s not part of JavaScript today – it’s a proposal for the future… Time to grow that brain! How might one, if so inclined, learn about these experimental JavaScript features and proposals?
Enter TC39 – “a group of JavaScript developers, implementers, academics, and more, collaborating with the community to maintain and evolve the definition of JavaScript” (from the official website). Cool. So, let’s take a peak at the stage 3 draft proposal for Array Grouping on Github.
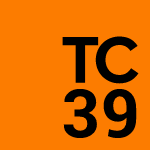
It’s from the proposal that a whole new world of experimentation was shown to me… Down at the bottom of the proposal, I notice a link to an external polyfill library – ‘core-js‘… Wait… Does this mean I get to play with the future of JavaScript today?! Yup!
This is all new to me, so I figured I should just start throwing stuff at the wall to see what sticks… Up first, let’s just install…
npm install --save core-js
Now let’s figure out how to use this bad boy… Since I have my Nodejs project setup as a module, I figure I will just try to import group. Note: I’m not saying this is the best way to go about this, or anything of the sort – just sharing my process of discovery in case there’s interest.
import group from 'core-js';
So far, so good.
Now, consider my project… It’s a basic utility script – imports a CSV, and then coerces the CSV data into JSON array containing objects that represent said data…
Here’s my CSV contents:
name;role;country
Sarene;Help Desk Operator;Thailand
Olvan;Nurse Practicioner;China
Janos;Cost Accountant;China
Dolph;Assistant Manager;China
Ariela;Database Administrator I;Azerbaijan
Lane;Environmental Tech;Indonesia
Griselda;Senior Quality Engineer;Portugal
Manda;Physical Therapy Assistant;Brazil
Leslie;Information Systems Manager;Japan
Aleen;Cost Accountant;Canada
Here’s my main coercion function and call to it (assume we’ve already read in and cleaned up our CSV):
function csvTxtToJsonArr(csvTxt, delimiter = ',') {
const headers = csvTxt.slice(0, csvTxt.indexOf('\n')).split(delimiter);
const rowStrs = csvTxt.slice(csvTxt.indexOf('\n') + 1).split('\n');
const jsonArr = rowStrs.map((currRowStr) => {
const rowVals = currRowStr.split(delimiter);
const mapObj = headers.reduce((newObj, currHeader, idx) => {
newObj[currHeader] = rowVals[idx];
return newObj;
}, {});
return mapObj;
});
return jsonArr
}
const jsonArr = csvTxtToJsonArr(csvContents, ';');
Which results in a jsonArr that looks like this:
[
{ name: 'Sarene', role: 'Help Desk Operator', country: 'Thailand' },
{ name: 'Olvan', role: 'Nurse Practicioner', country: 'China' },
{ name: 'Janos', role: 'Cost Accountant', country: 'China' },
{ name: 'Dolph', role: 'Assistant Manager', country: 'China' },
{
name: 'Ariela',
role: 'Database Administrator I',
country: 'Azerbaijan'
},
{ name: 'Lane', role: 'Environmental Tech', country: 'Indonesia' },
{
name: 'Griselda',
role: 'Senior Quality Engineer',
country: 'Portugal'
},
{
name: 'Manda',
role: 'Physical Therapy Assistant',
country: 'Brazil'
},
{
name: 'Leslie',
role: 'Information Systems Manager',
country: 'Japan'
},
{ name: 'Aleen', role: 'Cost Accountant', country: 'Canada' }
]
Cool… I guess… I mean, just basic, boring CSV play… But group – yeah, Array.prototype.group() seems like it might be fun here… So, let’s try it out using country as a grouping element:
jsonArr.group(({country}) => country);
Which gives us the following results:
{
Thailand: [
{ name: 'Sarene', role: 'Help Desk Operator', country: 'Thailand' }
],
China: [
{ name: 'Olvan', role: 'Nurse Practicioner', country: 'China' },
{ name: 'Janos', role: 'Cost Accountant', country: 'China' },
{ name: 'Dolph', role: 'Assistant Manager', country: 'China' }
],
Azerbaijan: [
{
name: 'Ariela',
role: 'Database Administrator I',
country: 'Azerbaijan'
}
],
Indonesia: [
{ name: 'Lane', role: 'Environmental Tech', country: 'Indonesia' }
],
Portugal: [
{
name: 'Griselda',
role: 'Senior Quality Engineer',
country: 'Portugal'
}
],
Brazil: [
{
name: 'Manda',
role: 'Physical Therapy Assistant',
country: 'Brazil'
}
],
Japan: [
{
name: 'Leslie',
role: 'Information Systems Manager',
country: 'Japan'
}
],
Canada: [ { name: 'Aleen', role: 'Cost Accountant', country: 'Canada' } ]
}
Cool! Right?!
Will Array.prototype.group() become standard spec? I can’t say, but it looks promising… But I suppose what I wanted to share more than this cool new group proposal was how you and I can be a little more hands on with what’s potentially to come to the future of JavaScript by way of the handy-dandy core-js library!
I feel that it’s worth mentioning another interesting and useful resource I ran across while playing this morning… The unofficial ES Proposals site, which is a labor of love from Apple Software Engineer Saad Quadri. Check it out!
Anywhos… Happy coding!
-Matt
Leave a Reply