I was today years old when I learned of the BroadcastChannel Web API, which facilitates intercommunication between windows, tabs, frames and iframes on the same domain (thanks to Cory House on Twitter).
At a glance, the API is surprisingly simple, so I figured why not give it a spin.
After consulting the ever-valuable MDN Web Docs on the topic, I had a BroadcastChannel spun up in a matter of seconds – here’s the overview and demonstration of it in action.
To setup my playground, I simply opened up a new Chrome window – and then 2 tabs, which I pointed to my blog here. At this point, all that’s left to be done is to new up an instance of BroadcastChannel in both tabs, setup an onmessage event listener in the receiver tab, and then to broadcast a message from the sender tab.
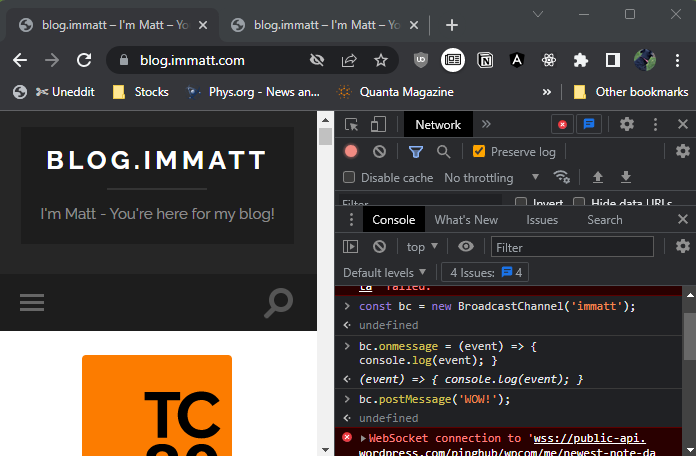
Note: We could have skipped setting up the listener here, but I wanted to demonstrate that the sending tab doesn’t display the posted message.
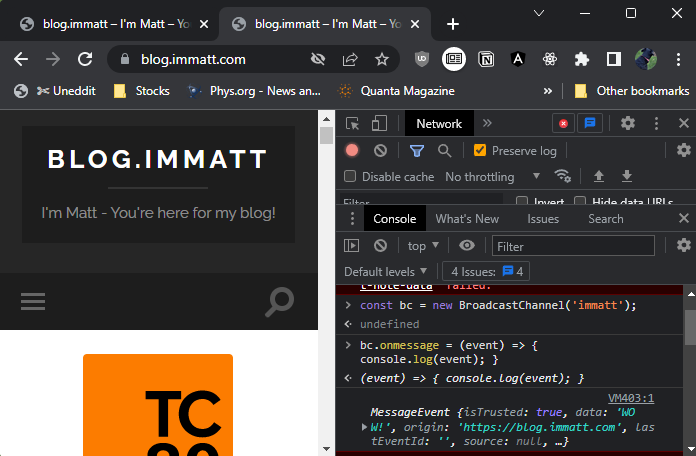
Note: The ‘WOW!’ message was sent from tab 1 after setting up the listener in tab 2 – previously posted messages from other tabs are not recieved in tabs/windows/frames instantiated after the message has been broadcast.
Cool, cool, cool. So pretty simple – here’s the explicit flow of this experiment in case it’s not clear from the screenshot captions:
- Open 2 tabs on the same domain – ‘https://blog.immatt.com’ in my example
- In tab 1, create a new BroadcastChannel instance via “new BroadcastChannel(‘channel-name’);”
- In tab 2, also create a new BroadcastChannel instance for the same channel name – e.g. “new BroadcastChannel(‘channel-name’);”
- In tab 2, setup an event listener for the ‘onmessage’ event exposed on our BroadcastChannel instance
- Back in tab 1, broadcast a message to all subscribers of our channel by using the ‘postMessage()’ function exposed on the ‘sender’ instance of BroadcastChannel
- Check back in tab 2 to see our super awesome message!
Here’s the expanded console object of the recieved message for a better look at the MessageEvent payload:
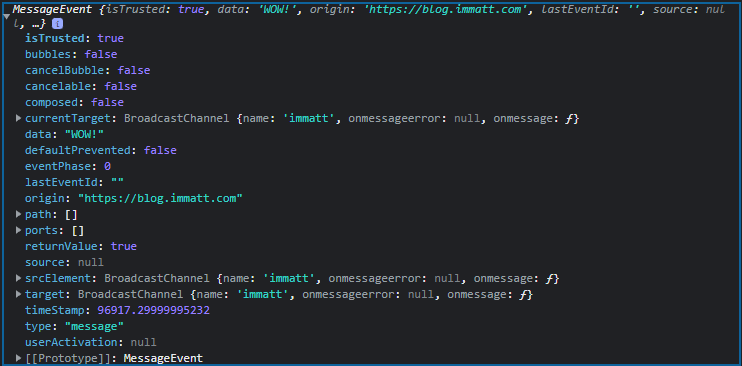
Also worth mentioning, browser compatibility is pretty solid – with it having landed in most major browsers around 2015/2016 (except Edge, which was 2020).
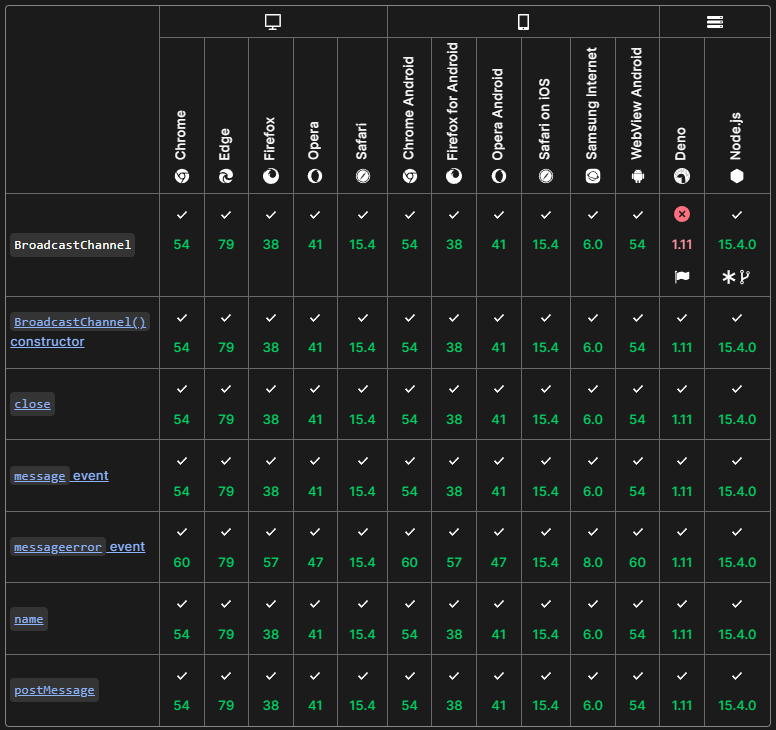
Anywhos. There you have it… Inter-tab/window/frame/iframe communications via the BroadcastChannel Web API!
Happy Coding!
-Matt