As a follow up to my last post, I’ve decided to dig in a little more to some of the upcoming TC39 proposals. The proposal that we’re reviewing today provides a few new ways to work with Arrays in an immutable way – a proposal titled “Change Array by copy”.
As the title “Change Array by copy” suggests, the new methods covered by the proposal provide Array functions which allow working with arrays in an immutable fashion (with the assumed intent of reducing side effects often associated with mutable objects, which are capable of being directly changed).
Included under the proposal are:
- Array.prototype.toSorted()
- Array.prototype.toReversed()
- Array.prototype.toSpliced()
- Array.prototype.with()
You can check out the collaborative view of this proposal on GitHub.
Let’s dive in!
Similarly to Array.prototype.sort(), Array.prototype.toSorted() exposes a helper function capable of sorting members of an array sequentially. Unlike to Array.protptype.sort(), Array.prototype.toSorted() returns a new array, by copy, leaving the original array untouched. To see this in action, see the following example:
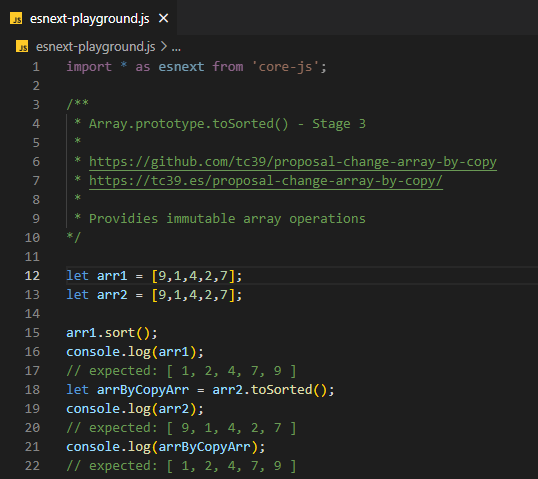
Up next, we have Array.prototype.toReversed() – essentially, the immutable form of Array.prototype.reverse(). Just as reverse() reverses the member order of the array, so to does toReversed() – but, again, without modifying the source array being operated on/against.
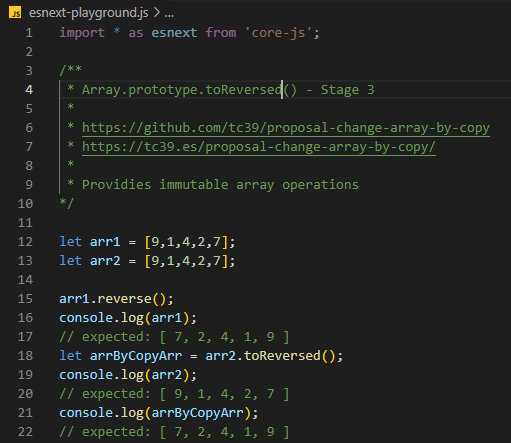
Last in our one-to-one function comparison is Array.prototype.toSpliced() – similar to the long standing and familiar Array.prototype.splice(), which is used to remove or replace elements within an array. As we can see in the following example, we’re capable of removing and replacing array elements with both splice() and toSpliced() – with the distinction that toSpliced() doesn’t modify the original array, and instead provides us a new, updated one via copy.
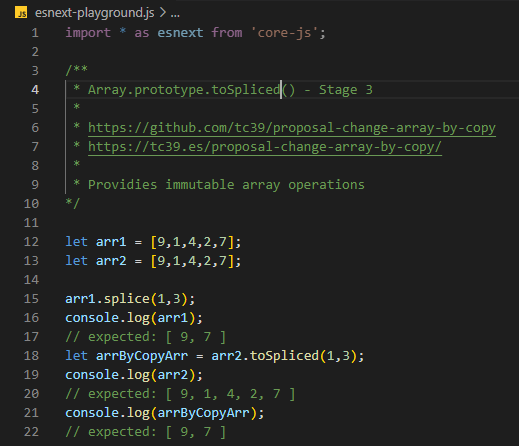
And finally, we have Array.prototype.with(). Unlike the previously discussed parts of the proposal, with() doesn’t really have a similarly named existing function – though we’re all likely familiar with the logic in play. When working with an array, it’s often common practice to reassign a value within an array by assigning a new value using the target’s zero-based index – with() exposes exactly this functionality, via a function call. See the following example to see Array.prototype.with() in play:
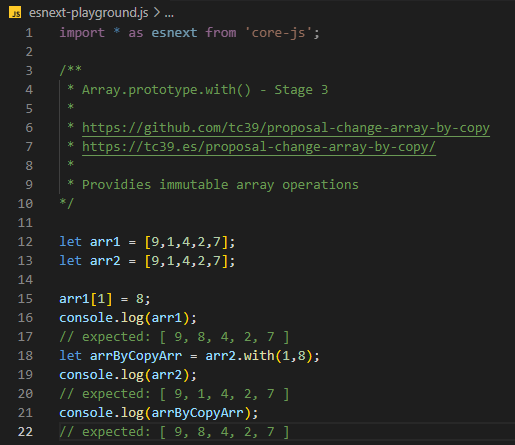
As you may have noticed in the above (and in case you did not) we’re once again using the core-js library to play with these new, not-yet-spec, JavaScript proposals – so check it out, and perhaps give it a spin!
Anywhos… I’m really enjoying having the opportunity to look forward and actually play with new stuff in the works for JavaScript. Yay, funemployment! That said, if you or someone you know is seeking a developer with well over a decade of full stack experience, including large enterprise engineering and architecture, please do reach out via matt@immmatt.com or ping me on Twitter @mattezell!
Happy Coding!
-Matt
Leave a Reply